主要參考官網這篇Create a Model and Database Table
1.db設定檔
指令:zf configure db-adapter
以下各別為開發、測試及成品設定其db
設定完後的結果會存在application/configs/application.ini
所以...其實到application.ini改就可以
那些指令那記得起來...
完整的檔案如下
2.建立table物件
針對table(guestbook)建立對應table物件Guestbook.php
指令:% zf create db-table Guestbook guestbook
Creating a DbTable at application/models/DbTable/Guestbook.php
Updating project profile 'zfproject.xml'
Guestbook.php的內容如下
3.建立Mapper
剛建立了table物件,在這要建立mapper
mapper的做用在處理insert,delete相關動作地方(要select的欄位,要下的where)
讓table物件與DB處理分開只負責存屬性(欄位)及方法,參考Data Mapper的觀念
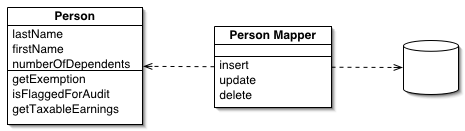
Creating a model at application/models/GuestbookMapper.php
Updating project profile '.zfproject.xml'
4.建立model
針對Guestbook建立該model
指令:% zf create model Guestbook
這指令會建立application/models/Guestbook.php
並更新project profile '.zfproject.xml'
5.建立controller
指令:% zf create controller Guestbook
=>會建立預設的index action
6.編輯view
總算結束了
鍵入http://localhost/guestbook 應該會看到下面畫面

1.db設定檔
指令:zf configure db-adapter
以下各別為開發、測試及成品設定其db
//設定成品db % zf configure db-adapter 'adapter=PDO_MYSQL&dbname="db_production"&host="localhost"&username="username"&password="ok1234"' production //設定測試db % zf configure db-adapter 'adapter=PDO_MYSQL&dbname="db_testing"' testing //設定開發db % zf configure db-adapter 'adapter=PDO_MYSQL&dbname="db_development"' development
所以...其實到application.ini改就可以
那些指令那記得起來...
完整的檔案如下
//application/configs/application.ini [production] phpSettings.display_startup_errors = 0 phpSettings.display_errors = 0 includePaths.library = APPLICATION_PATH "/../library" bootstrap.path = APPLICATION_PATH "/Bootstrap.php" bootstrap.class = "Bootstrap" appnamespace = "Application" resources.frontController.controllerDirectory = APPLICATION_PATH "/controllers" resources.frontController.params.displayExceptions = 0 resources.layout.layoutPath = APPLICATION_PATH "/layouts/scripts/" resources.view[]= resources.db.adapter = "PDO_MYSQL" resources.db.params.host = "localhost" resources.db.params.username = "root" resources.db.params.password = "ok1234" resources.db.params.dbname = "db_production" resources.db.params.driver_options.1002 = "SET NAMES utf8" [staging : production] [testing : production] phpSettings.display_startup_errors = 1 phpSettings.display_errors = 1 resources.db.adapter = "PDO_MYSQL" resources.db.params.dbname = "db_testing" [development : production] phpSettings.display_startup_errors = 1 phpSettings.display_errors = 1 resources.frontController.params.displayExceptions = 1 resources.db.adapter = "PDO_MYSQL" resources.db.params.dbname = "db_development"
中文亂碼問題
到application.ini中加入resources.db.params.driver_options.1002 = "SET NAMES utf8"
多個db問題
Zend_Application_Resource_Multidb
留著原本預設的db,才不用再改原本預設的資料
2.建立table物件
針對table(guestbook)建立對應table物件Guestbook.php
指令:% zf create db-table Guestbook guestbook
Creating a DbTable at application/models/DbTable/Guestbook.php
Updating project profile 'zfproject.xml'
Guestbook.php的內容如下
// application/models/DbTable/Guestbook.php /** * This is the DbTable class for the guestbook table. */ class Application_Model_DbTable_Guestbook extends Zend_Db_Table_Abstract { /** Table name */ protected $_name = 'guestbook'; }
3.建立Mapper
剛建立了table物件,在這要建立mapper
mapper的做用在處理insert,delete相關動作地方(要select的欄位,要下的where)
讓table物件與DB處理分開只負責存屬性(欄位)及方法,參考Data Mapper的觀念
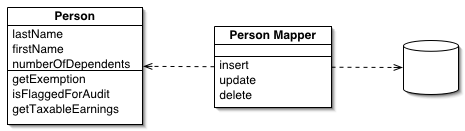
% zf create model GuestbookMapper
Updating project profile '.zfproject.xml'
// application/models/GuestbookMapper.php public function save($model); public function find($id, $model); public function fetchAll();
針對Guestbook建立該model
指令:% zf create model Guestbook
這指令會建立application/models/Guestbook.php
並更新project profile '.zfproject.xml'
// application/models/Guestbook.php class Application_Model_Guestbook { protected $_comment; protected $_created; protected $_email; protected $_id; public function __construct(array $options = null) { if (is_array($options)) { $this->setOptions($options); } } public function __set($name, $value) { $method = 'set' . $name; if (('mapper' == $name) || !method_exists($this, $method)) { throw new Exception('Invalid guestbook property'); } $this->$method($value); } public function __get($name) { $method = 'get' . $name; if (('mapper' == $name) || !method_exists($this, $method)) { throw new Exception('Invalid guestbook property'); } return $this->$method(); } public function setOptions(array $options) { $methods = get_class_methods($this); foreach ($options as $key => $value) { $method = 'set' . ucfirst($key); if (in_array($method, $methods)) { $this->$method($value); } } return $this; } public function setComment($text) { $this->_comment = (string) $text; return $this; } public function getComment() { return $this->_comment; } public function setEmail($email) { $this->_email = (string) $email; return $this; } public function getEmail() { return $this->_email; } public function setCreated($ts) { $this->_created = $ts; return $this; } public function getCreated() { return $this->_created; } public function setId($id) { $this->_id = (int) $id; return $this; } public function getId() { return $this->_id; } }
5.建立controller
指令:% zf create controller Guestbook
- Creating a controller at application/controllers/GuestbookController.php
- Creating an index action method in controller Guestbook
- Creating a view script for the index action method at application/views/scripts/guestbook/index.phtml
- Creating a controller test file at tests/application/controllers/GuestbookControllerTest.php
- Updating project profile '.zfproject.xml'
=>會建立預設的index action
// application/controllers/GuestbookController.php class GuestbookController extends Zend_Controller_Action { public function indexAction() { $guestbook = new Application_Model_GuestbookMapper(); $this->view->entries = $guestbook->fetchAll(); } }
6.編輯view
<!-- application/views/scripts/guestbook/index.phtml --> <p><a href="<?php echo $this->url( array( 'controller' => 'guestbook', 'action' => 'sign' ), 'default', true) ?>">Sign Our Guestbook</a></p> Guestbook Entries: <br /> <dl> <?php foreach ($this->entries as $entry): ?> <dt><?php echo $this->escape($entry->email) ?></dt> <dd><?php echo $this->escape($entry->comment) ?></dd> <?php endforeach ?> </dl>
總算結束了
鍵入http://localhost/guestbook 應該會看到下面畫面

沒有留言:
張貼留言